-
Notifications
You must be signed in to change notification settings - Fork 85
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
feat: add a new module stateless-prompt
- Loading branch information
Showing
9 changed files
with
202 additions
and
1 deletion.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,57 @@ | ||
# VK-IO Scenes | ||
<a href="https://www.npmjs.com/package/@vk-io/stateless-prompt"><img src="https://img.shields.io/npm/v/@vk-io/stateless-prompt.svg?style=flat-square" alt="NPM version"></a> | ||
<a href="https://github.com/negezor/vk-io/actions/workflows/tests.yml"><img src="https://img.shields.io/github/workflow/status/negezor/vk-io/VK-IO CI?style=flat-square" alt="Build Status"></a> | ||
<a href="https://www.npmjs.com/package/@vk-io/stateless-prompt"><img src="https://img.shields.io/npm/dt/@vk-io/stateless-prompt.svg?style=flat-square" alt="NPM downloads"></a> | ||
<a href="https://www.codacy.com/app/negezor/vk-io"><img src="https://img.shields.io/codacy/grade/25ee36d46e6e498981a74f8b0653aacc.svg?style=flat-square" alt="Code quality"></a> | ||
|
||
VK-IO Stateless Prompt - Simple implementation of middleware-based stateless prompt | ||
|
||
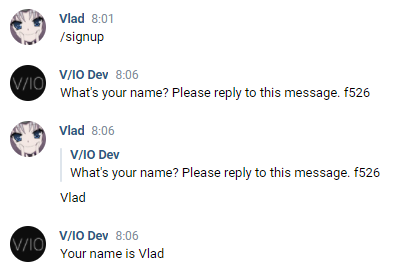 | ||
|
||
## Installation | ||
> **[Node.js](https://nodejs.org/) 12.0.0 or newer is required** | ||
### Yarn | ||
Recommended | ||
``` | ||
yarn add @vk-io/stateless-prompt | ||
``` | ||
|
||
### NPM | ||
``` | ||
npm i @vk-io/stateless-prompt | ||
``` | ||
|
||
## Example usage | ||
```js | ||
import { VK } from 'vk-io'; | ||
|
||
import { StatelessPromptManager } from '@vk-io/stateless-prompt'; | ||
|
||
const vk = new VK({ | ||
token: process.env.TOKEN | ||
}); | ||
|
||
const namePrompt = new StatelessPromptManager({ | ||
slug: 'name', | ||
handler: (context, next) => { | ||
if (!context.text) { | ||
return context.send('Please reply your name with text to previous message'); | ||
} | ||
|
||
return context.send(`Your name is ${context.text}`); | ||
} | ||
}); | ||
|
||
vk.updates.on('message_new', namePrompt.middlewareIntercept); | ||
|
||
vk.updates.on('message_new', (context, next) => { | ||
if (context.text === '/signup') { | ||
return context.send('What\'s your name? Please reply to this message. ' + namePrompt.suffix); | ||
} | ||
|
||
return next(); | ||
}); | ||
|
||
vk.updates.start().catch(console.error); | ||
``` |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,42 @@ | ||
{ | ||
"name": "@vk-io/stateless-prompt", | ||
"version": "1.0.0", | ||
"description": "Stateless prompts for the library vk-io", | ||
"license": "MIT", | ||
"author": { | ||
"name": "Vladlen (Negezor)", | ||
"email": "[email protected]" | ||
}, | ||
"repository": { | ||
"type": "git", | ||
"url": "git+https://github.com/negezor/vk-io.git", | ||
"directory": "packages/stateless-prompt" | ||
}, | ||
"homepage": "https://github.com/negezor/vk-io#readme", | ||
"bugs": "https://github.com/negezor/vk-io/issues", | ||
"keywords": [ | ||
"vk", | ||
"io", | ||
"stateless", | ||
"prompt" | ||
], | ||
"files": [ | ||
"lib", | ||
"typings" | ||
], | ||
"main": "./lib/index.js", | ||
"types": "./lib/index.d.ts", | ||
"exports": { | ||
".": { | ||
"import": "./lib/index.mjs", | ||
"require": "./lib/index.js" | ||
} | ||
}, | ||
"sideEffects": false, | ||
"engines": { | ||
"node": ">=12.0.0" | ||
}, | ||
"peerDependencies": { | ||
"vk-io": "^4.0.0" | ||
} | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,9 @@ | ||
import { createHash } from 'crypto'; | ||
|
||
export const getDataHash = (data: string): string => ( | ||
createHash('shake256', { | ||
outputLength: 2 | ||
}) | ||
.update(data) | ||
.digest('hex') | ||
); |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,6 @@ | ||
export * from './types'; | ||
export * from './stateless-prompt.types'; | ||
|
||
export { StatelessPromptManager } from './stateless-prompt-manager'; | ||
|
||
export { getDataHash } from './identifier'; |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,51 @@ | ||
import { getDataHash } from './identifier'; | ||
|
||
import { MessageContext, Middleware, HandlerMiddleware } from './types'; | ||
import { IStatelessPromptManagerOptions } from './stateless-prompt.types'; | ||
|
||
export class StatelessPromptManager { | ||
protected slug: string; | ||
|
||
protected uniqueIdentifier: string; | ||
|
||
protected handler: HandlerMiddleware; | ||
|
||
public constructor({ slug, handler }: IStatelessPromptManagerOptions) { | ||
this.slug = slug; | ||
this.uniqueIdentifier = getDataHash(slug); | ||
|
||
this.handler = handler; | ||
} | ||
|
||
/** | ||
* The suffix to add to the end of the message. | ||
* Due to this, the bot understands who is being addressed. | ||
* | ||
* ```ts | ||
* context.send('How old are you? Please reply to this message. ' + agePrompt.suffix); | ||
* ``` | ||
*/ | ||
public get suffix(): string { | ||
return this.uniqueIdentifier; | ||
} | ||
|
||
/** | ||
* Returns the middleware for intercept | ||
* | ||
* ```ts | ||
* updates.on('message_new', agePrompt.middlewareIntercept); | ||
* ``` | ||
*/ | ||
// eslint-disable-next-line class-methods-use-this | ||
public get middlewareIntercept(): Middleware<MessageContext> { | ||
return (context: MessageContext, next: Function): unknown => { | ||
const { replyMessage } = context; | ||
|
||
if (!replyMessage?.text?.endsWith(this.uniqueIdentifier)) { | ||
return next(); | ||
} | ||
|
||
return this.handler(context, next); | ||
}; | ||
} | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,27 @@ | ||
import { HandlerMiddleware } from './types'; | ||
|
||
export interface IStatelessPromptManagerOptions { | ||
/** | ||
* The slug should be unique. | ||
* It depends on it that the bot will respond and recognize the request. | ||
*/ | ||
slug: string; | ||
|
||
/** | ||
* Handles the prompt | ||
* | ||
* ```ts | ||
* const namePrompt = new StatelessPromptManager({ | ||
* slug: 'prompt-name', | ||
* handler: (context, next) => { | ||
* if (!context.text) { | ||
* return context.send('Please reply your name with text to previous message'); | ||
* } | ||
* | ||
* return context.send(`Your name is ${context.text}`); | ||
* } | ||
* }); | ||
* ``` | ||
*/ | ||
handler: HandlerMiddleware; | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,7 @@ | ||
import { MessageContext } from 'vk-io'; | ||
|
||
export type Middleware<T> = (context: T, next: Function) => unknown; | ||
|
||
export type HandlerMiddleware = Middleware<MessageContext>; | ||
|
||
export { MessageContext }; |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters